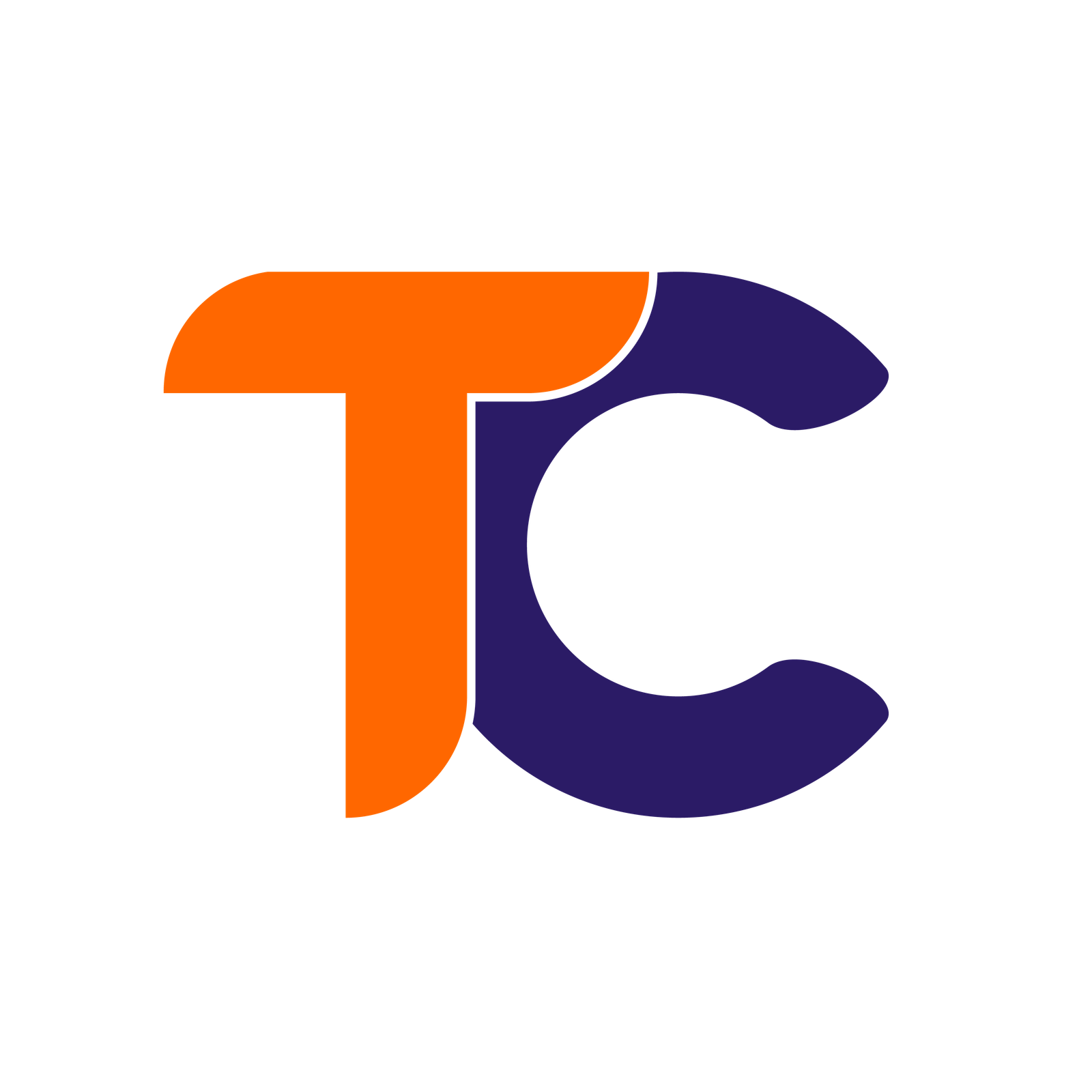
TechnoCruise
Motion Detection Using Python
Project Overview: In this project, you will develop a Python application that uses computer vision techniques to detect motion in a video stream or webcam feed. When motion is detected, the application will highlight the areas where motion is occurring, allowing users to monitor changes in their environment.
Tools and Libraries:
- Python
- OpenCV (Open Source Computer Vision Library)
Steps:
-
Setup:
- Install the required libraries using pip:
pip install opencv-python
.
- Install the required libraries using pip:
-
Capture Video Feed:
- Use OpenCV to access the webcam feed or load a video file as input.
- Initialize the camera capture object.
-
Initial Frame:
- Read the first frame from the video feed.
- Convert the frame to grayscale for better motion detection accuracy.
-
Motion Detection Loop:
- Create a loop to continuously process frames from the video feed.
- Read the next frame.
- Convert the frame to grayscale.
-
Frame Difference:
- Calculate the absolute difference between the current frame and the initial frame.
- Apply thresholding to the difference image to convert it into a binary image.
-
Contour Detection:
- Use the OpenCV
findContours
function to identify contours in the thresholded image. - Iterate through the detected contours and filter out small or irrelevant ones.
- Use the OpenCV
-
Motion Highlighting:
- Draw bounding boxes or rectangles around the significant contours found in the previous step.
- Use these rectangles to highlight the regions with detected motion on the original frame.
-
Display Output:
- Display the original frame with motion-highlighted areas in a window.
-
Termination:
- Add a way to exit the application, such as pressing the 'Esc' key.
Enhancements: Once you have a basic motion detection system working, you can consider adding more features and improvements to your project:
- Implement an alarm or notification system to alert users when motion is detected.
- Integrate a simple logging system to record timestamps when motion events occur.
- Experiment with more advanced motion detection algorithms, like background subtraction techniques.
- Develop a graphical user interface (GUI) to control the application and view historical motion events.
Learning Opportunities:
- Understanding computer vision basics.
- Handling video streams and frames in Python.
- Image differencing and thresholding.
- Working with contours and bounding boxes.
- Enhancing the user experience with GUI elements.
Remember that computer vision projects often involve trial and error, as well as fine-tuning parameters to achieve optimal results. Enjoy the process of learning and experimenting!
Struggling with your project build? We're here to assist.